Blubrry is a long standing provider of podcast hosting and IAB-certified statistics. They also offer a RESTful API for pulling podcast statistics, uploading new media, managing episodes and more. In this tutorial we will focus on the Blubrry Statistics/Analytics API and explore how you can integrate it in your own application through a practical, step-by-step example. (Full disclosure, I’m a software developer at Blubrry.)
This tutorial will cover the following:
- Using OAuth 2 for authentication and authorization
- Using Postman to make requests to the Blubrry API
- Integrating the API into a Python application
What can you do with the Blubrry Stats API?
The Blubrry Stats API allows you to pull information about your overall podcast downloads, as well as list individual episodes and their totals. By retrieving this data, you can perform your own data manipulation and calculations to further analyze trends within your show’s analytics. You can also create your own data visualization dashboard using a platform such as databox. Within databox, you can create dashboards showing your podcast stats, along with data from services like Google analytics, social media, and more all in one place. Learn more here.
Getting started
Since this tutorial will be using the Stats API, it assumes that you already have a show that uses Blubrry hosting or stats. If you are not using Blubrry services yet, you will first need to sign up for a free or paid plan. (Note that most of the API endpoints are only available with the paid plan).
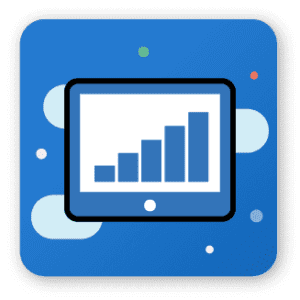
Analytics for your Podcast, regardless of where it's hosted
Even if your podcast is hosted elsewhere, you can still use the Blubrry statistics redirect to get data for your podcast. For only $5/month, you can get in-depth analytics on where your downloads are coming from and which platforms are most popular with your show. Click here to get one month free.
Blubrry API Keys
To access the API, you will need a client id and client secret from Blubrry. You can request keys here.
Using the Blubrry API in Postman
Postman is a tool that makes it easy to authenticate and test making requests to API endpoints.
This specification file contains all the Blubrry API endpoints available and will be imported into Postman so that we don’t have to manually type in every API call we want to make.
2. Import the OpenAPI specification file into Postman
Within Postman, on the top left hand corner click on Import. Click on Upload Files and select the OpenAPI file you downloaded in step 1. Leave the default settings and click import. The Blubrry API should appear as a collection on the left sidebar.
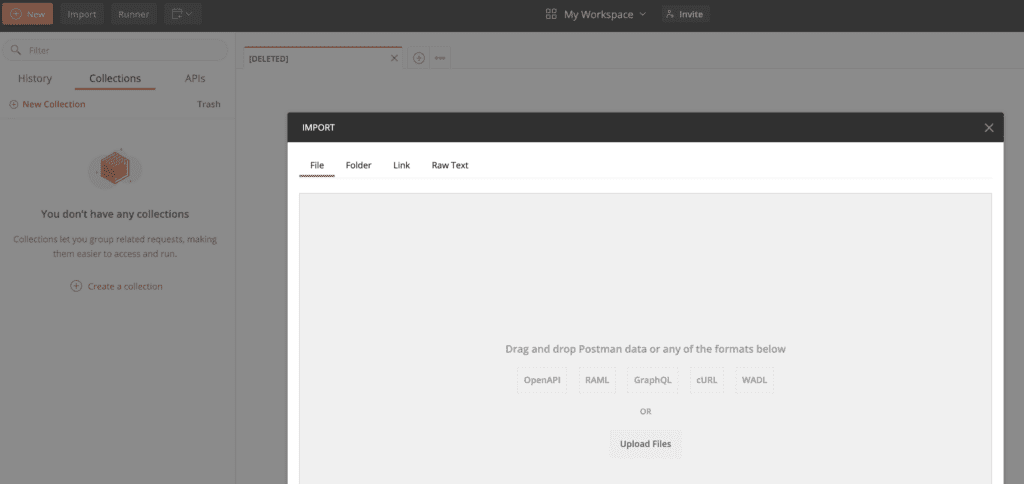
3. Setup OAuth 2.0 Authentication
In order to make API calls, we need to generate an access token using our Blubrry client id and secret. Edit the Blubrry API collection and go to the Authorization tab. Set the type to OAuth 2.0 and click on Get New Access Token.
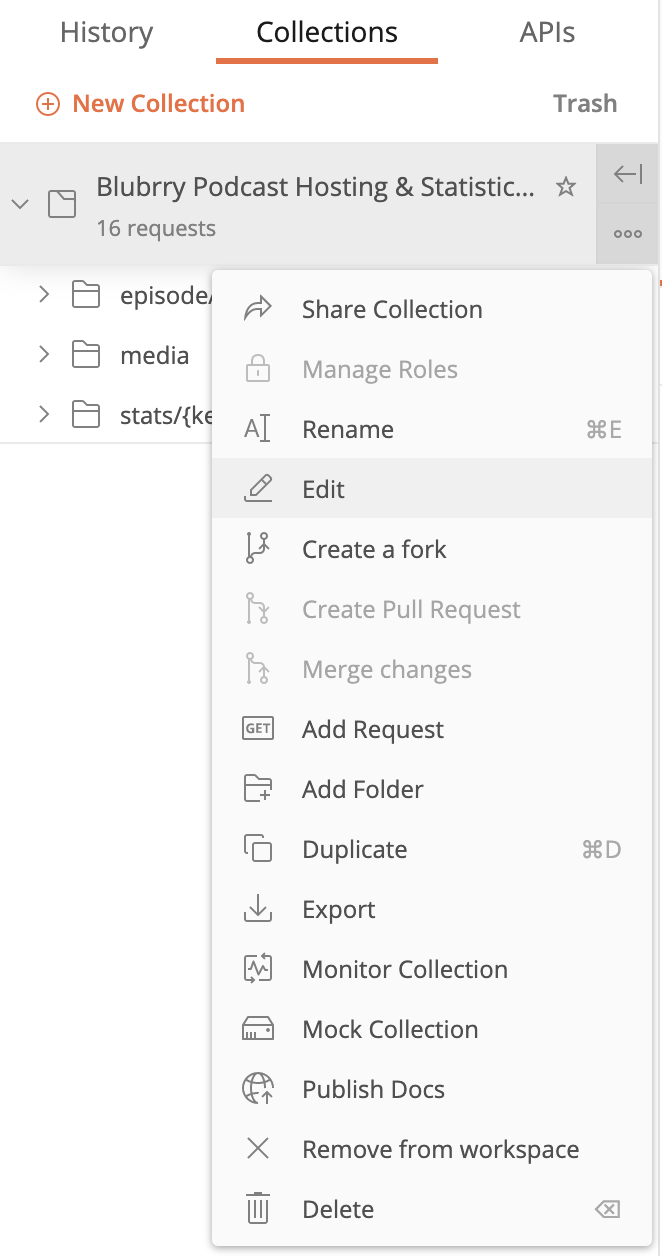
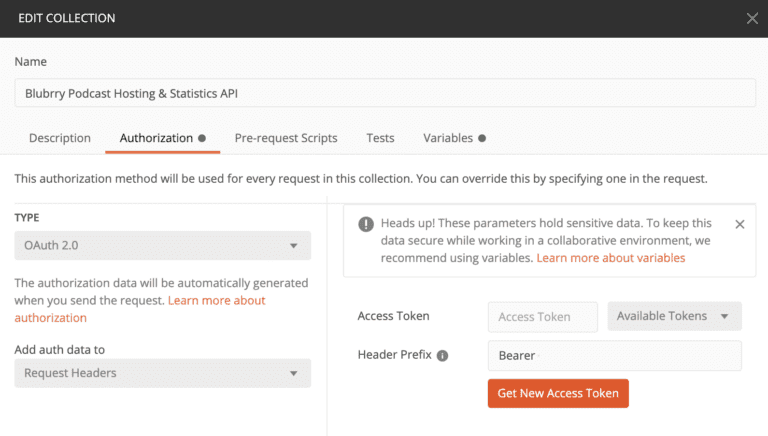
Get an access and refresh token
Fill in the fields as shown below, replacing the client id and secret fields with your API keys.
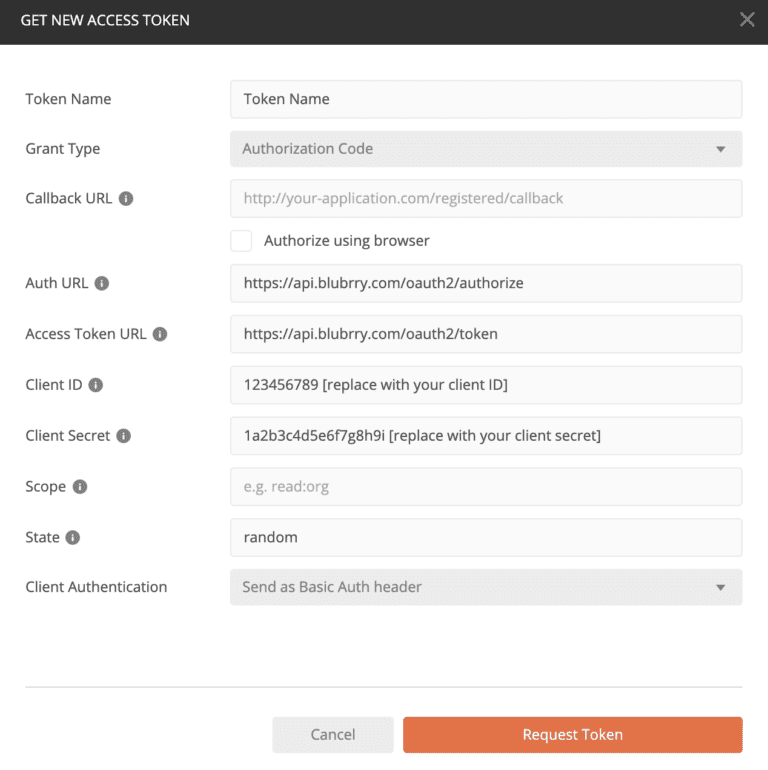
Once you click on Request Token, you will be asked to authenticate with your Blubrry account and grant access. If you are not signed in, it will first ask you to sign in with your Blubrry account email and password.
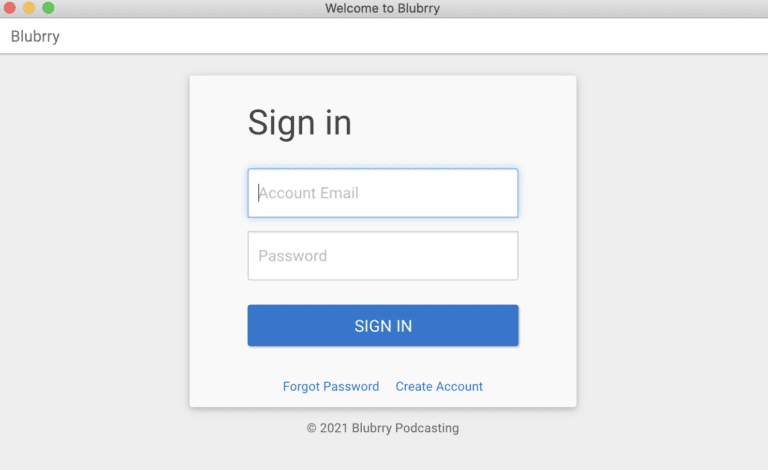
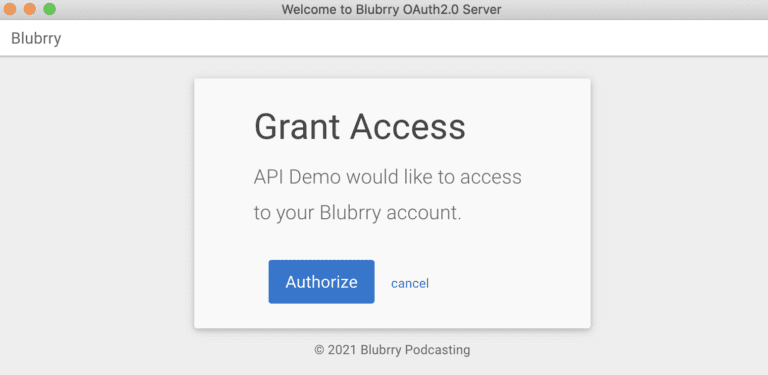
Upon granting access, you will receive an access token and a refresh token.
Access token: The access token needs to be included in the authorization header when making requests to all API endpoints. When making requests in Postman, the header will automatically be set for you. Access tokens expire 1 hour after being generated and will need to be regenerated using the refresh token for future requests.
Refresh token: The refresh token never expires and is therefore used to generate new access tokens. The refresh token should be stored securely and will be needed for integrating the API into your own custom application later.
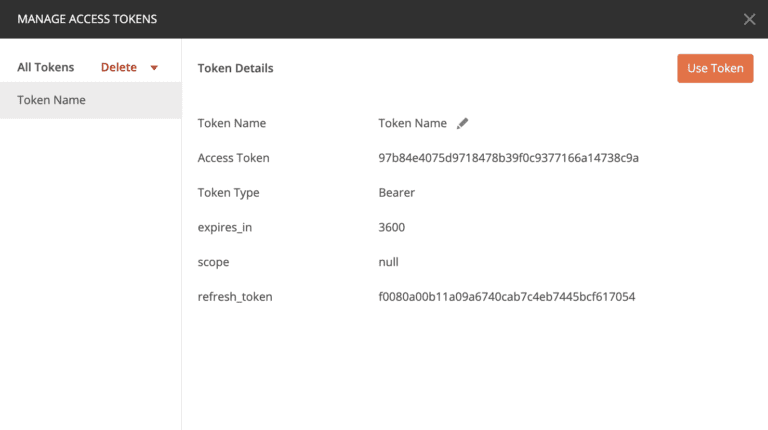
Make a request to the Blubrry statistics API endpoint
On the lefthand sidebar in Postman, select the stats folder. All the stats API endpoints will be listed. In this example, I made a request to the stats episodes endpoint. The request is shown in the grey bar and contains several variables:
- {{baseUrl}} – This variable will be set for you by default to https://api.blubrry.com/2/ (a variable defined in the collection settings ) and can be left as is.
- :keyword – This will automatically get substituted with the value you set in the path variable section of the Params tab. In the example, this is set to your_show_keyword. You should replace this with your show’s keyword.
- Start and limit parameters: These are optional and specify which range of episodes to return, in this case, episode 0-100.
Postman automatically uses the access token generated earlier to set the necessary authorization headers when making the API call. If it has been over an hour since you last generated an access token, you may need to generate a new one.
If the call is successful, you should get back some results. As shown below, in the case of the stats episodes endpoint, it should return a list of episodes and their download count, as well as some meta data.
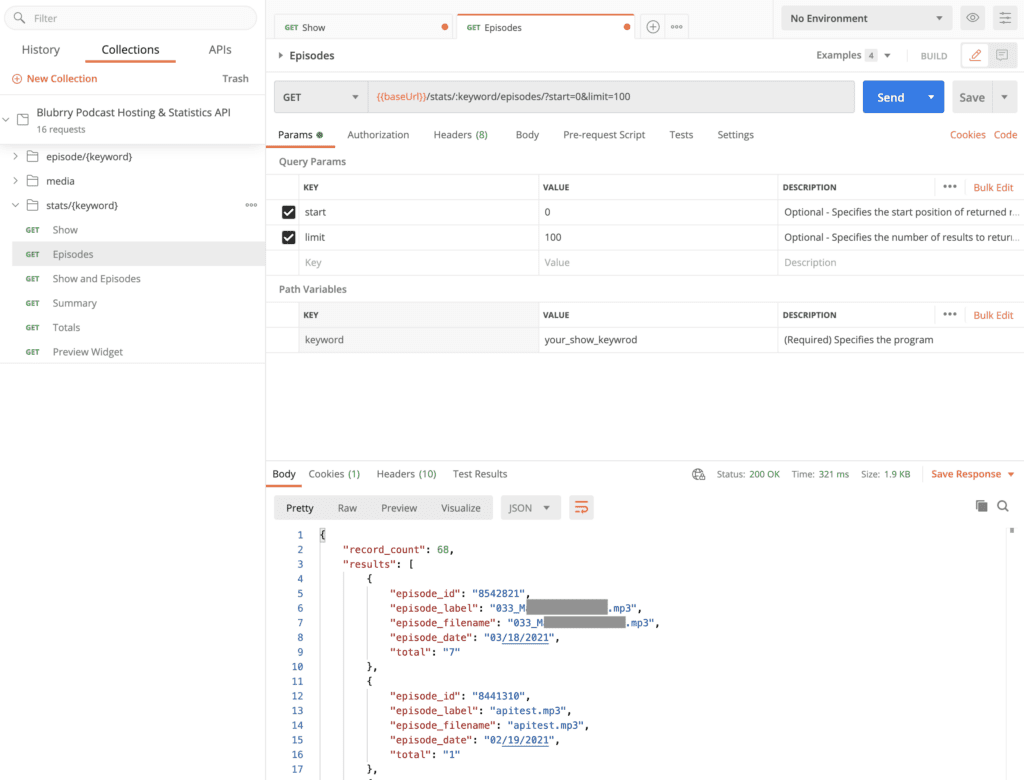
Integrating the Blubrry API into your Python application
Although we will be using python for this example, the process should be fairly similar in most programming languages. In order to make our api calls using python we need to:
- Define the credentials and request url.
- Use the refresh token to generate a new access token. (See step 3 of the Postman section of this tutorial for instructions on obtaining a refresh token).
- Use the access token to set the authorization header for the api call and make the request
- Get the result data
Below is a sample snippet of code in python with these steps implemented:
import requests
CLIENT_ID = 'XXXXX'
CLIENT_SECRET = 'XXXXX'
REFRESH_TOKEN = 'XXXXX'
REQUEST_URL = 'https://api.blubrry.com/2/stats/your_show_keyword/episodes/'
response = requests.post(
"https://api.blubrry.com/oauth2/token",
auth=(CLIENT_ID, CLIENT_SECRET),
data={
'grant_type': 'refresh_token',
'refresh_token': REFRESH_TOKEN
}
)
json_array = response.json()
access_token = json_array['access_token']
headers = {'Authorization': f'Bearer {access_token}'}
response = requests.get(REQUEST_URL, headers=headers)
results = response.content
Once you’re able to pull data, you can save it to a database to perform your own queries and analysis or push the data to a platform like databox to create data visualization dashboards.
You can adapt the code example to make calls to most of the other API endpoints provided by Blubrry. For the stats episodes endpoint from our example (/stats/keyword/episodes) we ran requests.get(…) because we are making a GET HTTP request. Some endpoints, such as the Blubrry media endpoints may require using the POST method instead. Refer to the Blubrry API documentation to check the HTTP method and specific parameters required for each endpoint.